Overriding getter/setter in TypeScript
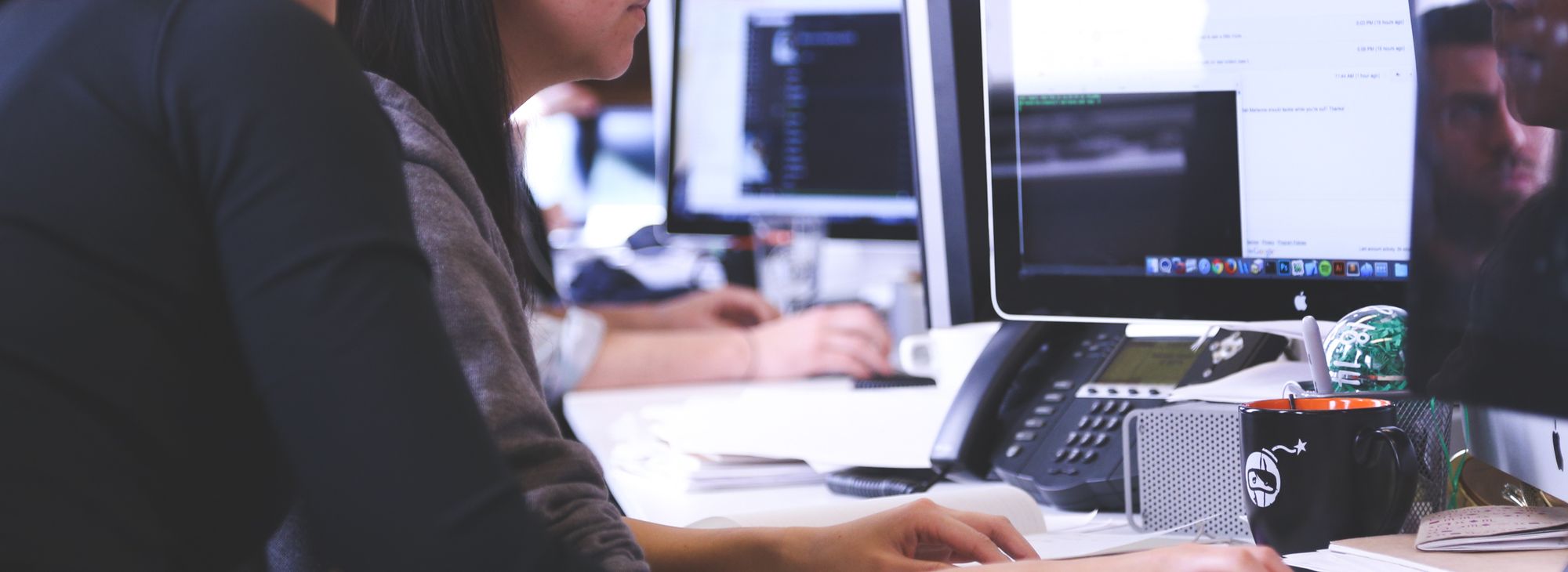
In contrast to the convention in Java, in TypeScript, we generally don't use getters or setters. We access and set variables directly. However, sometimes it is useful to perform some logic before a variable is set. TypeScript has a very neat feature to achieve that, without the need to refactor and putting method calls everywhere.
Suppose you have a variable age
:
export class Person {
private age: number;
constructor(){
this.age = -1; // This works :(
}
If you want to ensure that the age can only be set to realistic values, you can use the keyword set
in front of a method to make it behave like a variable.
export class Person {
private _age: number;
constructor(){
this.age = -1; // This now throws an exception :D
}
private set age(age: number){
if(this._age < 0){
throw new Error("Age is not valid.");
}
this._age = age;
}
}
I find this very useful when we accessing dates. Because the date picker we use returns an object, we have to use service to map the input to a string every time.
private get startDate(): string{
return this.dateService.fromDateInputObjToString(this._startDate);
}